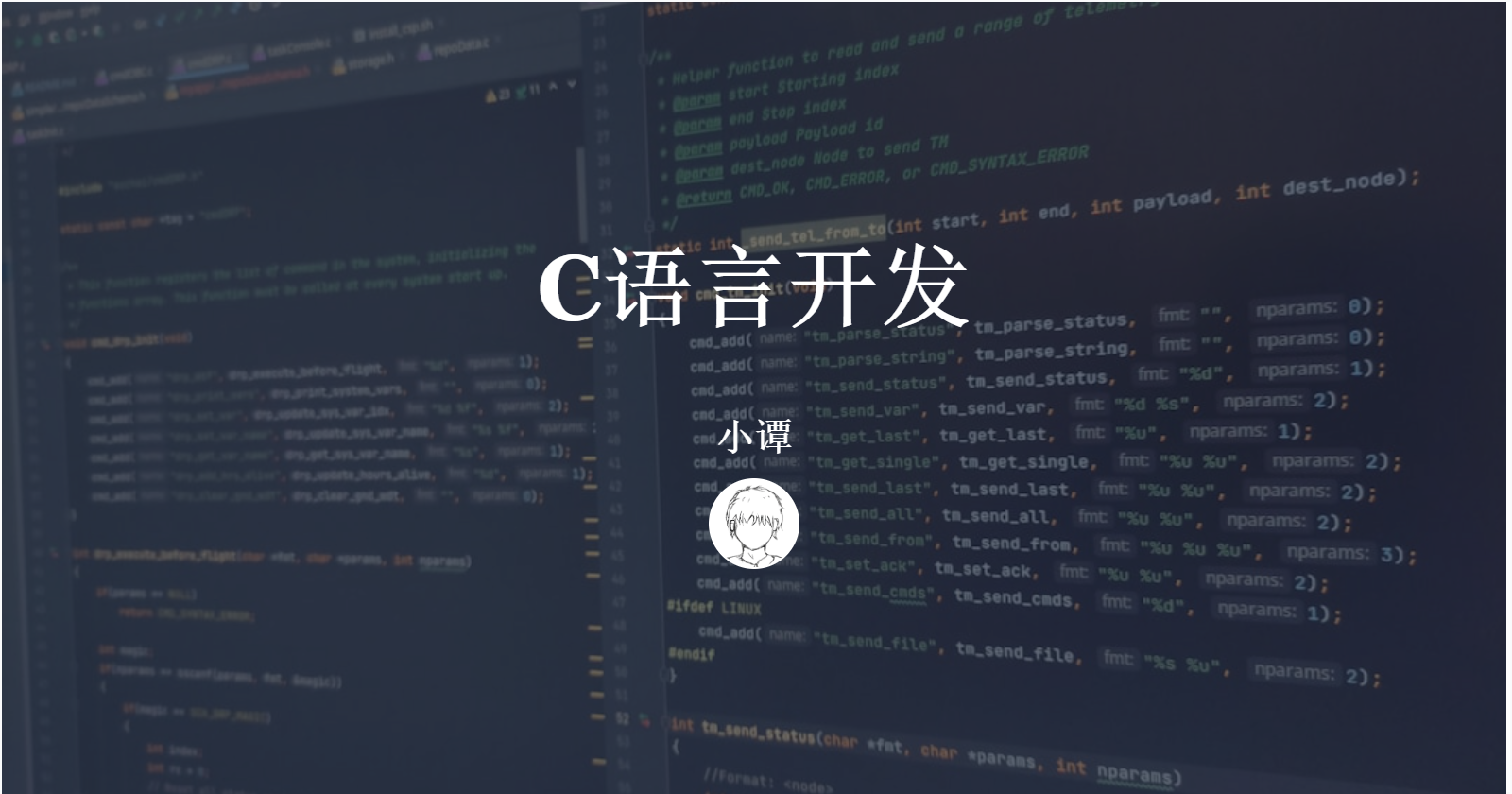
08_C语言-标准库
本文最后更新于 2025-03-18,学习久了要注意休息哟
第一章 时间库 - time.h
1.1 概述
-
time.h
是 C 标准库中提供的基础时间处理头文件,里面定义了时间相关的数据类型、宏与函数。 -
常见用途包括:
- 获取系统当前时间(即“Unix时间戳”),查看或记录事件发生时间。
- 格式化显示时间(如显示为
YYYY-MM-DD HH:MM:SS
)。 - 结构化处理年月日,如计算两个日期之间的差值等。
-
在大多数平台(包括 Linux、Windows、嵌入式等)都有实现,但在嵌入式系统中,要注意是否支持完整的操作系统时间功能。
提醒:如果需要更高精度计时或特定平台特性,还可查看对应系统API(如
clock_gettime()
、gettimeofday()
等)。
1.2 数据类型
:one: time_t
time_t
是最常见的时间类型,用来表示从某个参考时间点(通常是 1970-01-01 00:00:00 UTC,即 Unix Epoch)起过去的秒数。- 一般是一个长整数(long),但实际取决于系统和编译器,也有64位或32位的区别。
:two: struct tm
-
用来表示
分解后的时间
信息:年、月、日、小时、分钟、秒等。该结构通常定义如下:
struct tm { int tm_sec; // 秒 [0, 60] int tm_min; // 分 [0, 59] int tm_hour; // 小时 [0, 23] int tm_mday; // 一个月中的日期 [1, 31] int tm_mon; // 月份,从0开始计(0=1月, 11=12月) int tm_year; // 从1900年起计算的年数,如2025年则tm_year=125 int tm_wday; // 一周中的第几天 [0, 6] (0=周日) int tm_yday; // 一年中的第几天 [0, 365] int tm_isdst; // 夏令时标识 // 还有一些实现相关的扩展字段,视系统而定 };
-
通过
localtime()
/gmtime()
可以把time_t
转换为struct tm
,并可自行查看或修改年、月、日等细节。
:three: clock_t
clock_t
主要用于 CPU 占用时钟计数,配合clock()
函数来测量程序运行耗时(不是墙上时钟的时间,而是CPU的运行时间)。- 系统会用
CLOCKS_PER_SEC
宏定义表明“每秒钟对应多少个时钟计数”。
1.3 常用函数
:one: time(time_t *timer)
- 获取当前时间(秒级精度)并返回
time_t
值。 - 若参数
timer
非空,则将结果写入*timer
中;若为NULL
,则仅返回该值。
示例:获取当前时间并打印“秒数”
#include <stdio.h>
#include <time.h>
int main(void)
{
// 声明一个time_t变量,用于存储当前时间(秒数)
time_t now = time(NULL); // 等价于 time(&now);
if(now == (time_t)(-1))
{
// 如果返回 -1,表示获取时间失败
perror("获取当前时间失败");
return -1;
}
// 打印当前时间的数值(距1970-01-01 UTC的秒数)
printf("当前Unix时间戳: %ld\n", now);
return 0;
}
编译 & 运行
gcc example_time.c -o example_time
./example_time
输出示例
当前Unix时间戳: 1710678900 (示意值)
:two: localtime()
/ gmtime()
localtime(const time_t \*timer)
:将time_t
转换为本地时区的struct tm
。gmtime(const time_t \*timer)
:将time_t
转换为 UTC(世界协调时)时区的struct tm
。
#include <stdio.h>
#include <time.h>
int main(void)
{
time_t raw_time = time(NULL); // 获取当前时间(秒数)
if(raw_time == (time_t)(-1))
{
perror("无法获取当前时间");
return -1;
}
// 转为本地时间(结构体形式)
struct tm *local_time = localtime(&raw_time);
if(!local_time)
{
perror("localtime失败");
return -1;
}
// 打印结构体中的部分字段
printf("本地时间: %d年 %d月 %d日 %d:%d:%d\n",
local_time->tm_year + 1900, // 年要加1900
local_time->tm_mon + 1, // 月要加1
local_time->tm_mday,
local_time->tm_hour,
local_time->tm_min,
local_time->tm_sec
);
return 0;
}
示例输出(可能不同)
本地时间: 2025年 3月 18日 15:24:13
:three: asctime()
/ ctime()
asctime(struct tm \*timeptr)
:将分解的时间结构变为一个固定格式字符串 (形如"Wed Jan 2 02:03:55 1980\n"
)。ctime(time_t \*timer)
:结合time()
与asctime()
,直接把time_t
转为字符串(本地时区)。
#include <stdio.h>
#include <time.h>
int main(void)
{
time_t now = time(NULL);
// 1. 通过ctime获取字符串
char *time_str = ctime(&now);
if(time_str == NULL)
{
perror("ctime失败");
return -1;
}
printf("ctime字符串: %s", time_str); // 字符串自带换行
// 2. 如果需要更灵活的格式,可以用localtime + asctime
struct tm *info = localtime(&now);
char *asc_str = asctime(info);
printf("asctime字符串: %s", asc_str);
return 0;
}
输出示例
ctime字符串: Wed Mar 18 15:42:10 2025
asctime字符串: Wed Mar 18 15:42:10 2025
(注意 asctime()
、ctime()
返回的字符串末尾自带 \n
,并且这些函数一般返回静态缓冲区指针,使用时要小心线程安全和覆盖问题。)
:three:strftime()
- 可以自定义格式输出时间,如
YYYY-MM-DD HH:MM:SS
。 - 格式符常见示例:
%Y
:年份(如 2025)%m
:月份(01-12)%d
:日期(01-31)%H
:小时(00-23)%M
:分钟(00-59)%S
:秒(00-60)%a
/%A
:周几(英文缩写/完整拼写)- 其他更多参考文档
#include <stdio.h>
#include <time.h>
int main(void)
{
time_t now = time(NULL);
struct tm *local = localtime(&now);
char buffer[64];
// strftime(输出缓冲, 缓冲大小, 格式字符串, tm指针)
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", local);
printf("当前时间(自定义格式): %s\n", buffer);
return 0;
}
示例输出
当前时间(自定义格式): 2025-03-18 15:45:30
:five: 其他函数:mktime()
, difftime()
, clock()
等
mktime(struct tm \*timeptr)
:将struct tm
转回time_t
(以本地时间为基准)。可进行日期运算或修改后再转换。difftime(time_t end, time_t begin)
:计算两个time_t
之间相差的秒数,返回一个double
值。clock()
:返回自程序启动以来的 CPU 时钟计数,可结合CLOCKS_PER_SEC
转化为秒,常用于程序性能测量。
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 小道士